Building Beautiful UIs with Flutter: Tips and Tricks
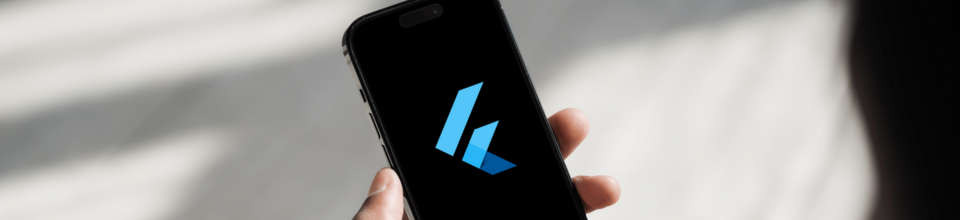
Flutter has revolutionized mobile app development by providing a powerful toolkit that simplifies the process of creating beautiful and functional UIs. One of the most compelling aspects of Flutter is its ease of use, especially for those familiar with JavaScript, as its syntax shares similarities. This makes it an attractive choice for developers who want to build stunning applications without delving into the complexities of native mobile app development.
In this post, we'll explore various techniques and best practices for designing visually appealing user interfaces using Flutter. We'll cover topics such as custom widgets, animations, theming, and responsive design, providing practical examples and code snippets along the way.
Custom Widgets: Building Blocks of Flutter UIs
Custom widgets are the heart of Flutter's UI design. By creating your own widgets, you can encapsulate functionality and design, making your code more reusable and maintainable. Here are a few tips for building custom widgets:
- Composition over Inheritance
Instead of extending existing widgets, try to compose new widgets by combining smaller widgets. This approach keeps your widgets simple and focused on a single responsibility.
Combining smaller widgets to create a reusable card UI, making the code cleaner and more maintainable.
- Leveraging InheritedWidget for State Sharing
InheritedWidget allows you to efficiently share state across your widget tree without resorting to complex state management solutions. This can be particularly useful for scenarios where you need to access shared data in multiple child widgets.
Using InheritedWidget to share the counter state across the widget tree, allowing efficient state management and access to shared data in multiple child widgets.
Animations: Bringing UIs to Life
Animations can make your app more engaging and enjoyable to use. Flutter provides a rich set of tools for creating animations.
- Implicit Animations
Implicit animations are easy to use and require minimal code changes. Use widgets like AnimatedContainer, AnimatedOpacity, and AnimatedPositioned to create smooth transitions.
Using AnimatedContainer to smoothly transition the size and color of the box when tapped, creating an engaging user experience. - Explicit Animations
For more complex animations, use the AnimationController and Tween classes. These provide fine-grained control over the animation's behavior.
Using AnimationController and Tween to create a continuous scaling animation, providing fine-grained control over the animation's behavior.
Theming: Consistent Look and Feel
Theming is crucial for maintaining a consistent look and feel across your app. Flutter's theming capabilities allow you to define colors, typography, and other design elements in a centralized way.
- Define a Theme
Create a theme by defining a ThemeData object and applying it to your MaterialApp.
Defining a custom theme and applying it to the entire app, ensuring a consistent look and feel. - Use the Theme
Access the theme properties using Theme.of(context).
Applying the custom theme to style the text, making use of the theme properties defined earlier.
Responsive Design: Adapting to Different Screen Sizes
Responsive design ensures that your app looks great on devices of all sizes. Flutter provides several tools to help you achieve this.
- LayoutBuilder
Use LayoutBuilder to build different layouts based on the available space.
Using LayoutBuilder to switch between a row and column layout based on screen width, ensuring the UI adapts to different screen sizes. - MediaQuery
Use MediaQuery to get information about the device's screen size and orientation.
Using MediaQuery to adjust text size based on the screen width, making the text responsive to different device sizes.
Conclusion
Building beautiful UIs with Flutter is both fun and rewarding. By leveraging custom widgets, animations, theming, and responsive design, you can create visually stunning and user-friendly applications. The examples and tips provided in this post should give you a solid foundation to start designing amazing UIs in Flutter. Happy coding!